this is some code thats free to use to get familar with coding
just run the code in python
print('A programming language is a set of commands, instructions, and other syntax use to create a software program. ... Many high-level languages are similar enough that programmers can easily understand source code written in multiple languages. Examples of high-level languages include C++, Java, Perl, and PHP.')
print(' ')
print(' ')
print('Java is a computer programming language. It enables programmers to write computer instructions using English-based commands instead of having to write in numeric codes.')
print(' ')
print(' ')
print('Python is an interpreted, object-oriented, high-level programming language with dynamic semantics. ... Python is simple, easy to learn syntax emphasizes readability and therefore reduces the cost of program maintenance. Python supports modules and packages, which encourages program modularity and code reuse.')
print(' ')
print(' ')
print('C++ is a programming language developed by Bjarne Stroustrup in 1979 at Bell Labs. C++ is regarded as a middle-level language, as it comprises a combination of both high-level and low-level language features. It is a superset of C, and that virtually any legal C program is a legal C++ program.')
print(' ')
print(' ')
print('JavaScript is a programming language commonly used in web development. It was originally developed by Netscape as a means to add dynamic and interactive elements to websites. Like server-side scripting languages, such as PHP and ASP, JavaScript code can be inserted anywhere within the HTML of a webpage.')
What is java?
Java is a computer programming language. It enables programmers to write computer instructions using English-based commands instead of having to write in numeric codes.

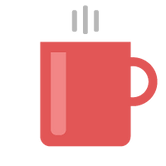

What is python?
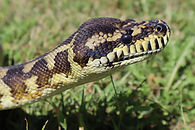
Python is an interpreted, object-oriented, high-level programming language with dynamic semantics. ... Python is simple, easy to learn syntax emphasizes readability and therefore reduces the cost of program maintenance. Python supports modules and packages, which encourages program modularity and code reuse.
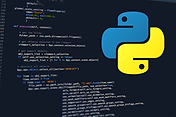
What is Java Script?
JavaScript is a programming language commonly used in web development. It was originally developed by Netscape as a means to add dynamic and interactive elements to websites. Like server-side scripting languages, such as PHP and ASP, JavaScript code can be inserted anywhere within the HTML of a webpage.
What is c++?
C++ is a programming language developed by Bjarne Stroustrup in 1979 at Bell Labs. C++ is regarded as a middle-level language, as it comprises a combination of both high-level and low-level language features. It is a superset of C, and that virtually any legal C program is a legal C++ program.

command_parse.py investment.py market_item.py
class CommandParse:
def __init__(self, dev=False):
self.commands =[]
self.dev = dev
def add_command(self, key, method, description, dev=False):
self.commands.append(Command(key, method, description, dev))
def parse(self, string):
string = string.lower()
segments = string.split(' ')
for i in self.commands:
# check for key match
if segments[0] == i.key:
# check for permissions
if i.dev is False or (i.dev is True and self.dev is True):
return i.method
return None
def list(self):
msg = ""
for i in self.commands:
if i.dev is False or (i.dev is True and self.dev is True):
temp = "\t`{}` - {}\n".format(i.key, i.description)
msg += temp
return msg
class Command:
def __init__(self, key, method, description, dev):
self.key = key
self.method = method
self.description = description
self.dev = dev
class Investment:
def __init__(self, value, marketID, current_value, marketItem):
"""
Constructor
:param value: the initial value invested
:param marketID: the id
:param current_value: the value the post is at at the time of investing
:param marketItem: The market object
"""
self.value = value
self.id = marketID
self.original_value = current_value
self.marketItem = marketItem
def get_value(self):
return round((self.value / self.original_value) * self.marketItem.value * 100) / 100
def cash_out(self):
self.marketItem.downvote(self.get_value())
class MarketItem:
def __init__(self, value, message):
self.value = value
self.id = message.id
self.message = message
def sub2pewds(self, amount=10000):
self.value -= 0
if self.value < 0:
self.value = 0
def remove_downvote(self, amount=6000):
self.value += amount
(to create items add
def ITEM NAME(self, amount=AMOUNT IT COSTS):
self.value -= 0
if self.value < 0:
self.value = 0
memeuser.py
class MemeUser:
def __init__(self, id, balance, name="Null"):
self.balance = balance
self.ID = id
self.name = name
self.investments = []
self.default_invest = 1000
self.bankrupt_count = 0
def add_investment(self, id, cur_value, marketItem, amount=None):
"""
Create an investment object or add value to an existing one
:param id: The ID of the post to invest in
:param cur_value: The current value of the post
:param marketItem: The post object
:param amount: The amount to invest in the post
:return: The success of the action (boolean)
"""
from investment import Investment
if amount is None:
amount = self.default_invest
# Check if the user has enough to invest
if self.balance <= 0:
return None
elif self.balance < amount:
amount = self.balance
self.balance -= amount
investmnt = Investment(amount, int(id), cur_value + amount, marketItem)
self.investments.append(investmnt)
marketItem.value += amount
# return True if an investment was successfully added
return True
def sell_all(self):
income = 0
for i in self.investments:
income += self.sell(i.id)
return income
def bankrupt(self, new_bal):
self.bankrupt_count += 1
self.investments = []
self.balance = new_bal
def sell(self, invst):
# find the investment in the list by its ID
for i in self.investments:
if i.id == invst:
invst = i
break
# if no investment is found with that ID
return None
# remove from investment list
self.investments.remove(invst)
# calculate earnings before cashing out
income = invst.get_value()
self.balance += income
invst.cash_out()
return income
def set_defaults(self, invest):
self.default_invest = invest
def get_investment(self, iid):
for i in self.investments:
if i.id == iid:
return i
return None
def get_investment_display(self):
# compose lines
result = []
for i in self.investments:
result.append("Post id: " + str(i.id))
result.append("Initially invested: $" + str(i.value))
val = i.get_value() # current investment val calculated a share %
result.append("current Value: $" + str(val))
result.append("----------------------------------")
# compose string
output = ""
for i in result:
output += i
output += '\n'
# don't return an empty message
if output != "":
return output
else:
return None
def get_outstanding(self):
temp = 0
for i in self.investments:
temp += i.get_value()
return temp
import discord
import argparse
from market_item import MarketItem
from memeuser import MemeUser
from command_parse import CommandParse
parser = argparse.ArgumentParser(description='Discord bot to facilitate investing in posts.')
# required arguments
parser.add_argument('-t', '--token', help='your discord bot token', required=True)
parser.add_argument('-c', '--channel', help='the channel ID designated for investing', required=True)
# optional arguments
parser.add_argument('--init_balance', help='the balance that users start at initially', required=False, default=200)
parser.add_argument('--init_post', help='the balance that posts start at. Higher makes returns lower', required=False, default=1000)
parser.add_argument('-d', '--dev', help='enables some developer commands', required=False, default=False, action='store_true')
args = parser.parse_args()
TOKEN = args.token
channelID = int(args.channel)
client = discord.Client()
cparse = CommandParse(args.dev)
# global values
userlist = []
initbalance = int(args.init_balance)
init_post_balance = int(args.init_post)
activeMarkets = []
memechannel = None
skim_percent = 0.02
# TRIGGERED FUNCTIONS
@client.event
async def on_ready():
print('Logged in as')
print(client.user.name)
print(client.user.id)
print('------------')
# Load some required information and cache in memory
global memechannel
global userlist
memechannel = client.get_channel(channelID)
if memechannel is not None:
print("Meme channel detected")
else:
print("Channel not detected")
exit(420)
potentialUsers = memechannel.guild.members
for m in potentialUsers:
if m != client.user and await meme_user_exists(m.id) is False:
nu = MemeUser(m.id, initbalance, m.name)
userlist.append(nu)
print("{} users detected and processed".format(len(userlist)))
print("User starting balance: ${}".format(initbalance))
if args.dev is True:
print("Starting Bot in DEVELOPER MODE")
print("------------")
@client.event
async def on_member_join(member):
if await meme_user_exists(member.id) is False:
nu = MemeUser(member.id, initbalance, member.name)
userlist.append(nu)
print("New User added and given ${} in starting money".format(initbalance))
@client.event
async def on_message(message):
# we do not want the bot to reply to itself
if message.author == client.user:
return
# If the message is a DM it will check these conditions
if message.guild is None:
print(message.content)
method = cparse.parse(message.content)
if method is not None:
await method(message)
else: # The message is NOT a DM it will count it for investing if it is in the correct channel
# We add the up and downvote reactions and add the post to a list for counting
if message.channel.id == channelID:
await message.add_reaction('👍')
await message.add_reaction('👎')
mi = MarketItem(init_post_balance, message)
activeMarkets.append(mi)
# This function is called when a reaction is added to a message
# Only applies to messages sent AFTER the bot joined the server
@client.event
async def on_reaction_add(reaction, user):
# Ignore reactions in other channels and reactions made by the bot
if reaction.message.channel.id != channelID or user == client.user:
return
if reaction.emoji == '👍':
await create_investment(reaction.message, user)
elif reaction.emoji == '👎':
mi = await get_market_item(reaction.message.id)
mi.downvote()
@client.event
async def on_reaction_remove(reaction, user):
if reaction.emoji == '👎':
mi = await get_market_item(reaction.message.id)
mi.remove_downvote()
# DEVELOPER &/OR ADMIN FUNCTIONS
async def subtract(message):
"""
Developer function to subtract funds
"""
if args.dev is True:
user = await get_user(message.author.id)
m = message.content.split(' ')
# check second parameter
if len(m) == 1:
await message.channel.send("You must provide an integer value after the command.")
return None
user.balance -= int(m[1])
await message.channel.send("Your new balance is `${}`".format(user.balance))
async def add(message):
"""
Developer function to add funds
"""
if args.dev is True:
user = await get_user(message.author.id)
m = message.content.split(' ')
# check second parameter
if len(m) == 1:
await message.channel.send("You must provide an integer value after the command.")
return None
user.balance += int(m[1])
await message.channel.send("Your new balance is `${}`".format(user.balance))
# USER FUNCTIONS
async def get_help(message):
msg = "Hello {0.author.mention}, here are some things you can ask me: \n".format(message)
msg += cparse.list()
msg += "To invest in memes simply react to them with 👍\n"\
"When investing with 👍, you will always invest what your `default_investment` is set to."
await message.channel.send(msg)
async def change_default_investment(message):
m = message.content.split(' ')
if len(m) != 2:
await message.channel.send("Please ensure there is only a single number argument after the command.")
return None
try:
new_val = int(m[1])
except ValueError:
await message.channel.send("Sorry, that wasn't a number.")
return None
user = await get_user(message.author.id)
user.default_invest = new_val
await message.channel.send("Default Investment Value changed to `${}`".format(new_val))
async def check_balance(message):
userID = message.author.id
for u in userlist:
if u.ID == userID:
msg = "Here is your balance information:\n" \
"`Balance: ${}`\n" \
"`Total Investments Outstanding: ${}`\n" \
"`Default investment amount: ${}`".format(u.balance, u.get_outstanding(), u.default_invest)
await message.channel.send(msg)
return
async def create_investment(message, discord_user):
mi = await get_market_item(message.id)
meme_user = await get_user(discord_user.id)
if meme_user is None:
print("User does not exist")
print("this should probably not happen?")
return
else:
ret = meme_user.add_investment(message.id, mi.value, mi)
# ret is returned None if the investment is not added
if ret is None:
print("Investment not added, user insufficient funds")
return None
else:
if message.author.id != discord_user.id:
# the user who posted the meme gets a kickback for posting a dank meme
meme_poster = await get_user(message.author.id)
meme_poster.balance += int(meme_user.default_invest * skim_percent)
# if the user has not messaged the bot since it has started it first needs to create the DM channel
if discord_user.dm_channel is None:
await discord_user.create_dm()
# Then send the confirmation DM
await discord_user.dm_channel.send("You have successfully invested ${}".format(meme_user.default_invest))
async def show_investments(message):
uid = message.author.id
user = await get_user(uid)
msg = user.get_investment_display()
if msg is not None:
await message.channel.send(msg)
else:
await message.channel.send("You currently have `0` investments.")
await message.channel.send("You have declared bankruptcy {} times.".format(user.bankrupt_count))
async def sell(message):
m = message.content.split(' ')
user = await get_user(message.author.id)
if len(m) != 2:
await message.channel.send("Please ensure there is only a single number argument after the command.")
return None
elif m[1] == 'all':
income = user.sell_all()
else:
income = user.sell(int(m[1]))
if income is None:
await message.channel.send("You do not have any investments with that ID.")
else:
await message.channel.send("Income from sale: `${}`".format(income))
async def declare_bankruptcy(message):
user = await get_user(message.author.id)
# if the user has no outstanding investments and has less then a quarter of the starting value
if user.get_outstanding() == 0 and user.balance < initbalance / 4:
user.bankrupt(initbalance / 4)
await message.channel.send("You have successfully declared bankruptcy. You have been granted "
"an initial balance of ${}.".format(initbalance / 4))
elif user.get_outstanding() != 0:
await message.channel.send("You cannot declare bankruptcy unless you have 0 investments.")
else:
await message.channel.send("You have too much money to declare bankruptcy.")
async def get_leaderboards(message):
# sort the user list by their net worth
sorted_list = sorted(userlist, key=lambda x: x.balance + x.get_outstanding(), reverse=True)
msg = "__TOP {} USERS__\n".format(min(len(sorted_list), 5))
for m in range(min(len(sorted_list), 5)):
bal = sorted_list[m].balance + sorted_list[m].get_outstanding()
msg += "{}. {} : ${}\n".format(m + 1, sorted_list[m].name, bal)
await message.channel.send(msg)
# UTILITY FUNCTIONS
async def meme_user_exists(checkID):
for u in userlist:
if u.ID == checkID:
return True
return False
# TODO order userlist and use a better search method like quicksearch im just lazy. fine for small servers
async def get_user(uid):
for u in userlist:
if u.ID == uid:
return u
return None # this shouldn't happen
async def get_market_item(mid):
for m in activeMarkets:
if m.id == mid:
return m
return None
cparse.add_command('!balance', check_balance, "Check your current balance")
cparse.add_command('!help', get_help, "Display the available commands")
cparse.add_command('!portfolio', show_investments, "display all of your current investments")
cparse.add_command('!val', change_default_investment, "Change your default investment amount")
cparse.add_command('!sell', sell, "Cash out some investments. Use keyword 'all' to sell all at once or use a post ID")
cparse.add_command('!bankrupt', declare_bankruptcy, "Declare bankruptcy if you are close to $0")
cparse.add_command('!leaderboard', get_leaderboards, "Show the current leaderboard")
cparse.add_command('!subtract', subtract, "DEV - subtract from your current balance", dev=True)
cparse.add_command('!add', add, "DEV - add to your current balance", dev=True)
client.run('YOUR TOKEN HERE')
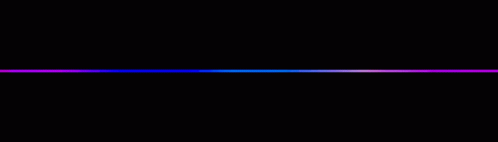
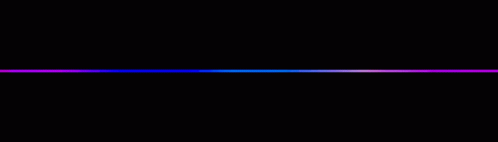
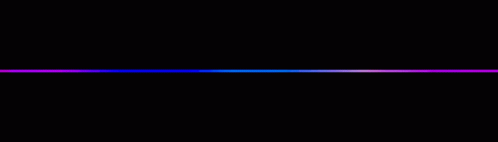
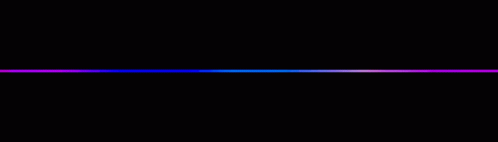
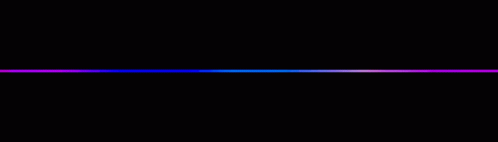
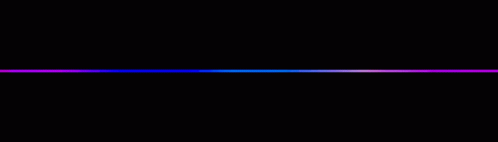
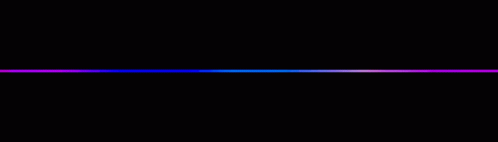
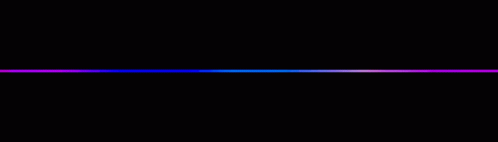
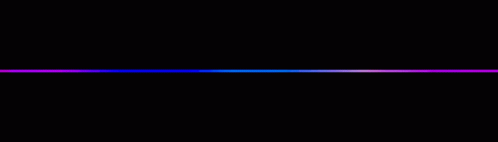
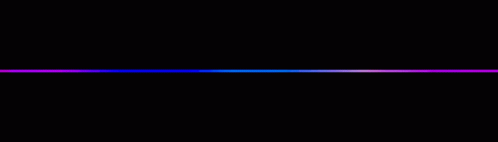
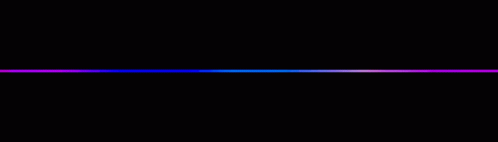
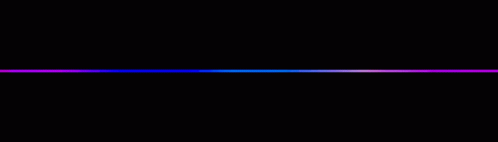
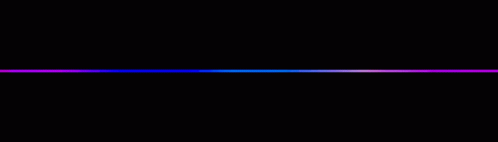
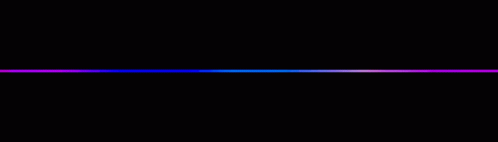
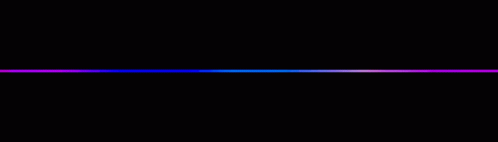
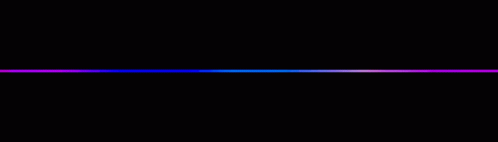